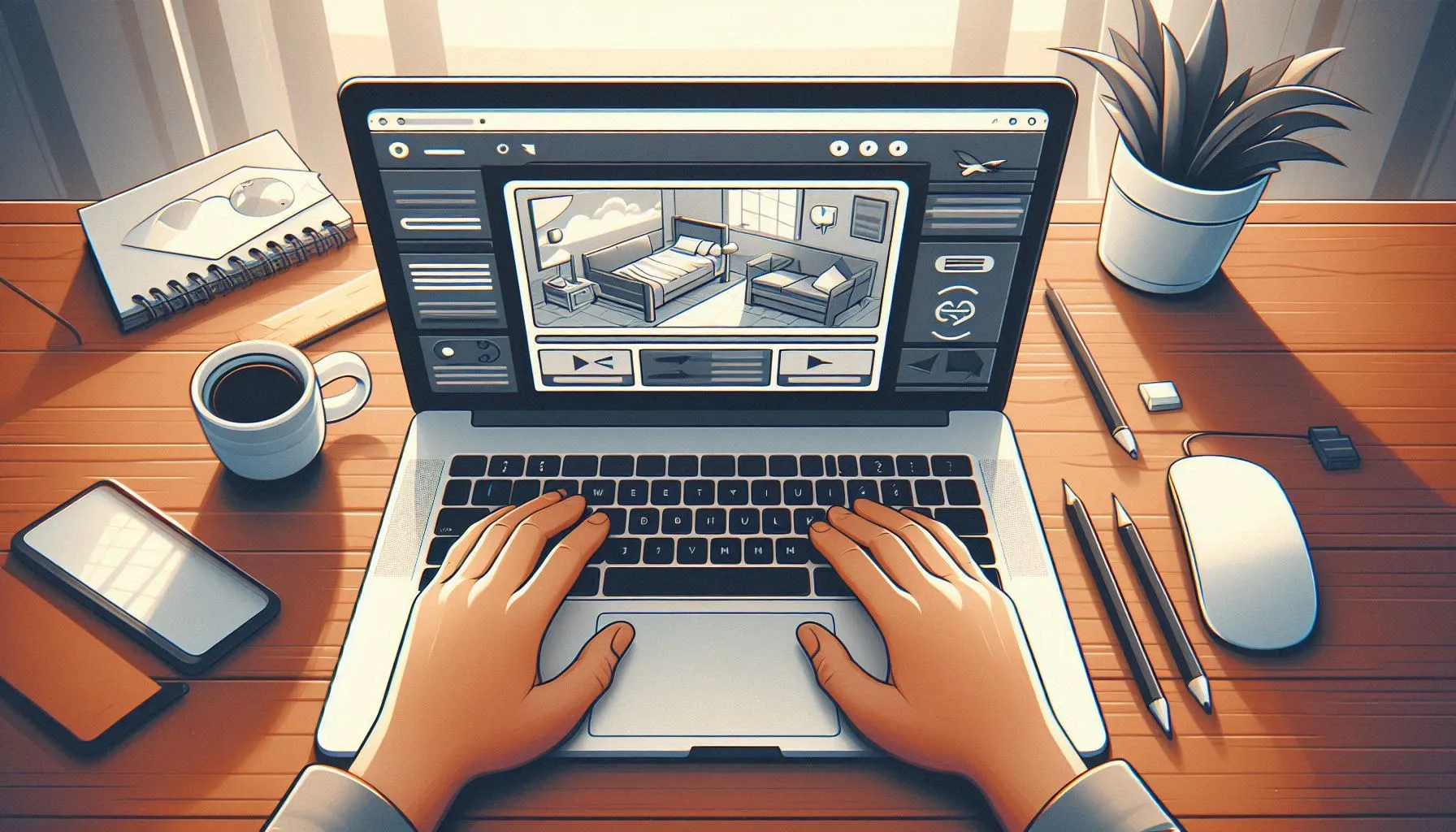
Horizontal scrolling
Create a Horizontal Scroll
● Introduction
I was looking for a subject to write my first post ever on this blog when I thought of my CodePen space, where I created a couple of effects over the past few years. The most interesting one is clearly the horizontal scrolling, which is what I’ll detail today. Let’s see how to replace the scrolling behavior of your website.
● Core Concept
It may seem hard, but it’s actually really simple. We have to do two things:
- Disable the default scroll wheel event.
- Create a new scroll wheel event.
The first point is very simple. When working with events in JavaScript, we can use methods such as preventDefault
, which disables the default behavior of an event.
For the second point, we just need to scroll the container (the one we want to modify) by the amount the user scrolls. This value is stored in the read-only deltaY
attribute of a WheelEvent
. This value is a number and is positive when the user scrolls down, and negative when scrolling up. We then need to apply this value to the container’s scrollLeft
attribute. This was the concept, now let’s turn it into code.
● Code
First, we need to retrieve the container. In my example, I use the main
tag as my container.
const container = document.querySelector("main");
Next, we add a new event listener with addEventListener
and specify the event type as “wheel”. (You can find a list of all possible event types here.)
container.addEventListener("wheel", (e) => {
});
Finally, as mentioned in the concept, disable the default behavior and apply the scroll delta to the container’s horizontal scroll.
container.addEventListener("wheel", (e) => {
e.preventDefault();
container.scrollLeft += e.deltaY;
});
● Result
Here is the code required to see the effect in action. I’ve added some styling to make it visible, but only the JavaScript code is strictly required:
- The
html
andbody
elements have no margin and a sans-serif font for cleaner styling. - The
main
tag is set todisplay: flex;
so that the sections inside it are arranged horizontally. - Each section takes up at least half of the viewport’s width (
min-width: 50vw
) and the full height (min-height: 100vh
), making the sections large enough to require scrolling. - Sections have centered text, and every second section gets a teal background with white text.
HTML
<main>
<section>
<h1>1</h1>
</section>
<section>
<h1>2</h1>
</section>
<section>
<h1>3</h1>
</section>
<section>
<h1>4</h1>
</section>
</main>
CSS
html, body {
margin: 0;
font-family: sans-serif;
}
main {
overflow-x: hidden;
display: flex;
}
section {
min-width: 50vw;
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
font-size: 4ch;
}
section:nth-child(even) {
background-color: teal;
color: white;
}
JavaScript
const scrollContainer = document.querySelector("main");
scrollContainer.addEventListener("wheel", (evt) => {
evt.preventDefault();
scrollContainer.scrollLeft += evt.deltaY;
});
● Conclusion
Although it is much simpler than it looks, this effect radically changes your website’s behavior, helping to differentiate it from others. I initially made this effect for my portfolio and haven’t changed it since. If you enjoyed this article, feel free to share it on your social media or read another one!